スポンサーリンク
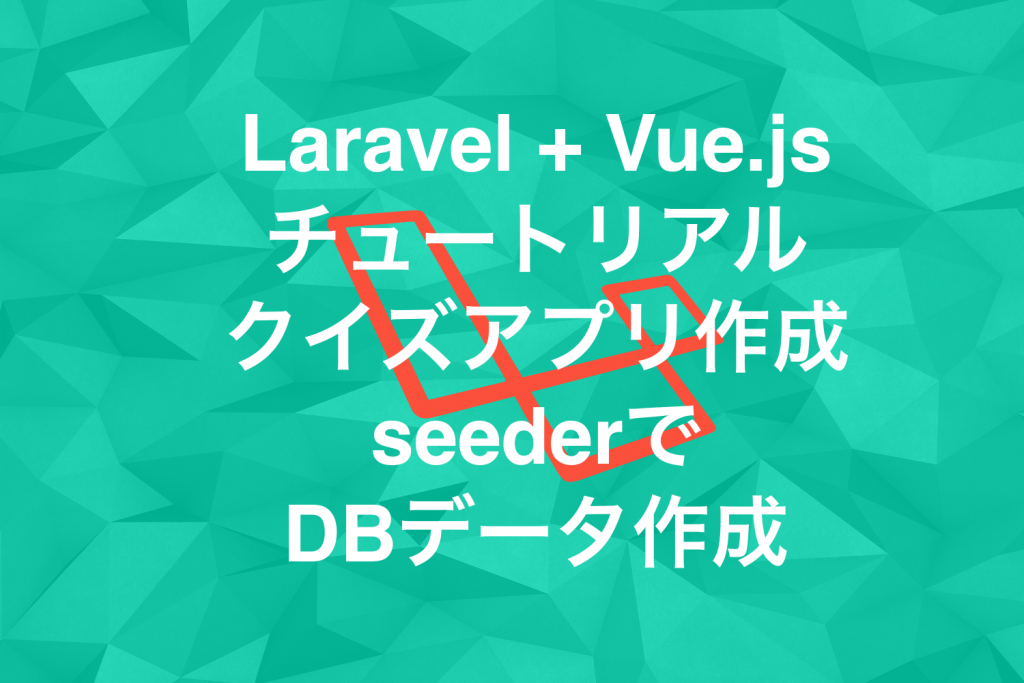
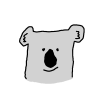
今回はseederでDBのデータを作成しよう
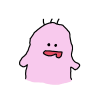
はい、先生!お願いします!!
この記事を書いた人
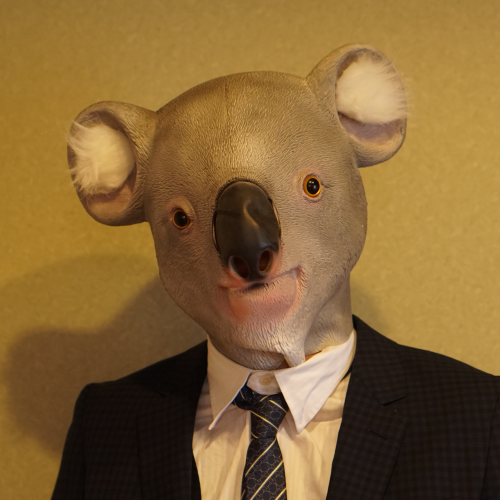
プロコアラ@エンジニアブロガー
10年間エンジニアをしており、副業でWebサイトやWebサービスを作っています。
一時期資格取得にハマりTOEIC860点オーバー、応用情報処理は取得。休日はラズパイをいじるコアラ好きです。
Follow @top_pro_koala
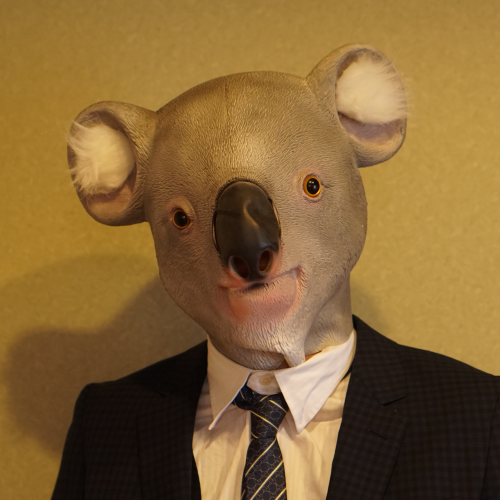
プロコアラ@エンジニアブロガー
10年間エンジニアをしており、副業でWebサイトやWebサービスを作っています。
一時期資格取得にハマりTOEIC860点オーバー、応用情報処理は取得。休日はラズパイをいじるコアラ好きです。
Follow @top_pro_koala
スポンサーリンク
今回のチュートリアル概要
今回は、データベースのデータ設定をコマンド一発でできるようになるseederを解説します。
Modelが中心のお話になります。
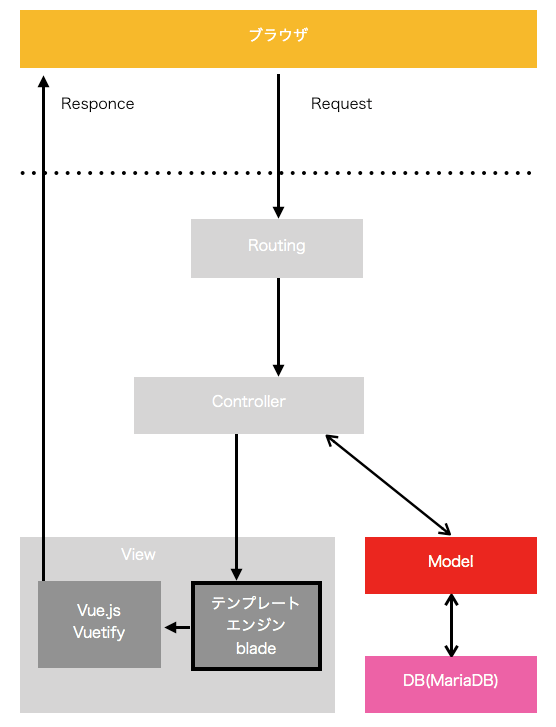
テスト一覧ページの修正
テスト一覧ページのリンクをクリックすると、個別のテスト詳細ページに移動するようにします。
以前作成しているtests_tableのSeederのマイグレーションファイルは以下の通りです。
<?php use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class CreateTestsTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('tests', function (Blueprint $table) { $table->bigIncrements('id'); $table->timestamps(); $table->string('test_name'); $table->date('test_day'); $table->integer('applicant')->nullable(); $table->integer('examinee')->nullable(); $table->integer('passed')->nullable(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('tests'); }
Seederファイルを次のコマンドを実行して作成します。
php artisan make:seeder TestSeeder
作成されたTestSeederファイルを修正します。
基本情報処理試験のデータをtestsテーブルに設定するようにします。
<?php use Illuminate\Database\Seeder; // use Carbon\Carbon; class TestSeeder extends Seeder { /** * Run the database seeds. * * @return void */ public function run() { // $now = Carbon::now(); DB::table('tests')->truncate(); DB::table('tests')->insert([ [ 'test_name' => '平成21年度春期試験', 'test_day' => '20090419', 'applicant' => '90752', 'examinee' => '64544', 'passed' => '17685', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成21年度秋期試験', 'test_day' => '20091018', 'applicant' => '107800', 'examinee' => '79829', 'passed' => '28270', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成22年度春期試験', 'test_day' => '20100418', 'applicant' => '92108', 'examinee' => '65407', 'passed' => '14489', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成22年度秋期試験', 'test_day' => '20101017', 'applicant' => '100113', 'examinee' => '73242', 'passed' => '17129', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成23年度特別試験', 'test_day' => '20110710', 'applicant' => '88001', 'examinee' => '58993', 'passed' => '14579', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成23年度秋期試験', 'test_day' => '20111016', 'applicant' => '82090', 'examinee' => '59505', 'passed' => '15569', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成24年度春期試験', 'test_day' => '20120415', 'applicant' => '75085', 'examinee' => '52582', 'passed' => '12437', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成24年度秋期試験', 'test_day' => '20121021', 'applicant' => '79674', 'examinee' => '58905', 'passed' => '15987', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成25年度春期試験', 'test_day' => '20130421', 'applicant' => '66667', 'examinee' => '46416', 'passed' => '10674', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成25年度秋期試験', 'test_day' => '20131020', 'applicant' => '76020', 'examinee' => '55426', 'passed' => '12274', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成26年度春季試験', 'test_day' => '20140420', 'applicant' => '65141', 'examinee' => '46005', 'passed' => '11003', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成26年度秋期試験', 'test_day' => '20141019', 'applicant' => '74577', 'examinee' => '54874', 'passed' => '12950', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成27年度春期試験', 'test_day' => '20150419', 'applicant' => '65570', 'examinee' => '46874', 'passed' => '12174', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成27年度秋期試験', 'test_day' => '20151018', 'applicant' => '73221', 'examinee' => '54347', 'passed' => '13935', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成28年度春期試験', 'test_day' => '20160417', 'applicant' => '61281', 'examinee' => '44184', 'passed' => '13418', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成28年度秋期試験', 'test_day' => '20161016', 'applicant' => '74095', 'examinee' => '55815', 'passed' => '13173', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成29年度春期試験', 'test_day' => '20170416', 'applicant' => '67784', 'examinee' => '48875', 'passed' => '10975', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成29年度秋期試験', 'test_day' => '20171015', 'applicant' => '76717', 'examinee' => '56377', 'passed' => '12313', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成30年度春期試験', 'test_day' => '20180415', 'applicant' => '73581', 'examinee' => '51377', 'passed' => '14829', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成30年度秋期試験', 'test_day' => '20181019', 'applicant' => '82347', 'examinee' => '60004', 'passed' => '13723', // 'created_at' => $now, // 'updated_at' => $now, ], [ 'test_name' => '平成31年度春期試験', 'test_day' => '20191017', 'applicant' => '77470', 'examinee' => '54686', 'passed' => '12155', // 'created_at' => $now, // 'updated_at' => $now, ], ]); } }
<template> <div class="container"> <v-layout> <v-flex> <v-card> <v-card-title> <h1>試験一覧</h1> </v-card-title> <v-card-text> <p><a href="/">Home</a> >> テスト一覧</p> <v-data-table :headers="headers" :items="tests" class="elevations-1" hide-actions > <template v-slot:items="props"> <tr> <td><a v-bind:href="props.item.id | add_path">{{props.item.test_name}}</a></td> <td>{{props.item.test_day}}</td> <td>{{props.item.applicant | number_format}}</td> <td>{{props.item.examinee | number_format}}</td> <td>{{props.item.passed | number_format}}</td> <td>{{props.item.examinee_rate}}</td> <td>{{props.item.passed_rate}}</td> </tr> </template> </v-data-table> </v-card-text> </v-card> </v-flex> </v-layout> </div> </template> <script> export default { props: ["tests"], data: () => ({ headers: [ {text:'試験名', value:'test_name'}, {text:'試験日', value:'test_day'}, {text:'応募者', value:'applicant'}, {text:'受験者', value:'examinee'}, {text:'合格者', value:'passed'}, {text:'受験率(%)', value:'examinee_rate'}, {text:'合格率(%)', value:'passed_rate'}, ] }), filters: { number_format(value) { if(!value) return '0' return value.toString().replace(/(\d)(?=(\d{3})+$)/g, '$1,') }, add_path(value) { return "/test/" + value.toString() } }, } </script>
Seederを実行するには次のコマンドを実行します。
php artisan db:seed
DBに設定されます。
MariaDB [homestead]> select * from tests; +----+------------+------------+----------------------------+------------+-----------+----------+--------+ | id | created_at | updated_at | test_name | test_day | applicant | examinee | passed | +----+------------+------------+----------------------------+------------+-----------+----------+--------+ | 1 | NULL | NULL | 平成21年度春期試験 | 2009-04-19 | 90752 | 64544 | 17685 | | 2 | NULL | NULL | 平成21年度秋期試験 | 2009-10-18 | 107800 | 79829 | 28270 | | 3 | NULL | NULL | 平成22年度春期試験 | 2010-04-18 | 92108 | 65407 | 14489 | | 4 | NULL | NULL | 平成22年度秋期試験 | 2010-10-17 | 100113 | 73242 | 17129 | | 5 | NULL | NULL | 平成23年度特別試験 | 2011-07-10 | 88001 | 58993 | 14579 | | 6 | NULL | NULL | 平成23年度秋期試験 | 2011-10-16 | 82090 | 59505 | 15569 | | 7 | NULL | NULL | 平成24年度春期試験 | 2012-04-15 | 75085 | 52582 | 12437 | | 8 | NULL | NULL | 平成24年度秋期試験 | 2012-10-21 | 79674 | 58905 | 15987 | | 9 | NULL | NULL | 平成25年度春期試験 | 2013-04-21 | 66667 | 46416 | 10674 | | 10 | NULL | NULL | 平成25年度秋期試験 | 2013-10-20 | 76020 | 55426 | 12274 | | 11 | NULL | NULL | 平成26年度春季試験 | 2014-04-20 | 65141 | 46005 | 11003 | | 12 | NULL | NULL | 平成26年度秋期試験 | 2014-10-19 | 74577 | 54874 | 12950 | | 13 | NULL | NULL | 平成27年度春期試験 | 2015-04-19 | 65570 | 46874 | 12174 | | 14 | NULL | NULL | 平成27年度秋期試験 | 2015-10-18 | 73221 | 54347 | 13935 | | 15 | NULL | NULL | 平成28年度春期試験 | 2016-04-17 | 61281 | 44184 | 13418 | | 16 | NULL | NULL | 平成28年度秋期試験 | 2016-10-16 | 74095 | 55815 | 13173 | | 17 | NULL | NULL | 平成29年度春期試験 | 2017-04-16 | 67784 | 48875 | 10975 | | 18 | NULL | NULL | 平成29年度秋期試験 | 2017-10-15 | 76717 | 56377 | 12313 | | 19 | NULL | NULL | 平成30年度春期試験 | 2018-04-15 | 73581 | 51377 | 14829 | | 20 | NULL | NULL | 平成30年度秋期試験 | 2018-10-19 | 82347 | 60004 | 13723 | | 21 | NULL | NULL | 平成31年度春期試験 | 2019-10-17 | 77470 | 54686 | 12155 | +----+------------+------------+----------------------------+------------+-----------+----------+--------+
まとめ
Seederを使用することによって、コマンド1つでDBにデータを設定することができるようになりました。
DBを使用することにより、検索して必要なデータを取得することができるようになります。
今回はここまで。ではでは!
スポンサーリンク
スポンサーリンク